In programming, algorithms play a crucial role in problem-solving and automation. One of the common types of algorithms used in Java involves printing patterns using nested loops. A popular pattern among beginners is the “star pattern,” which helps in understanding loops and conditional statements better. Let’s explore the Java star algorithm, its types, and how to implement them.
Understanding the Java Star Algorithm
A Java star algorithm refers to a pattern printing algorithm that uses loops to display stars (*
) in different shapes. These patterns help developers practice control flow, loops, and nested iterations. There are different types of star patterns, categorized based on their shapes and structures.
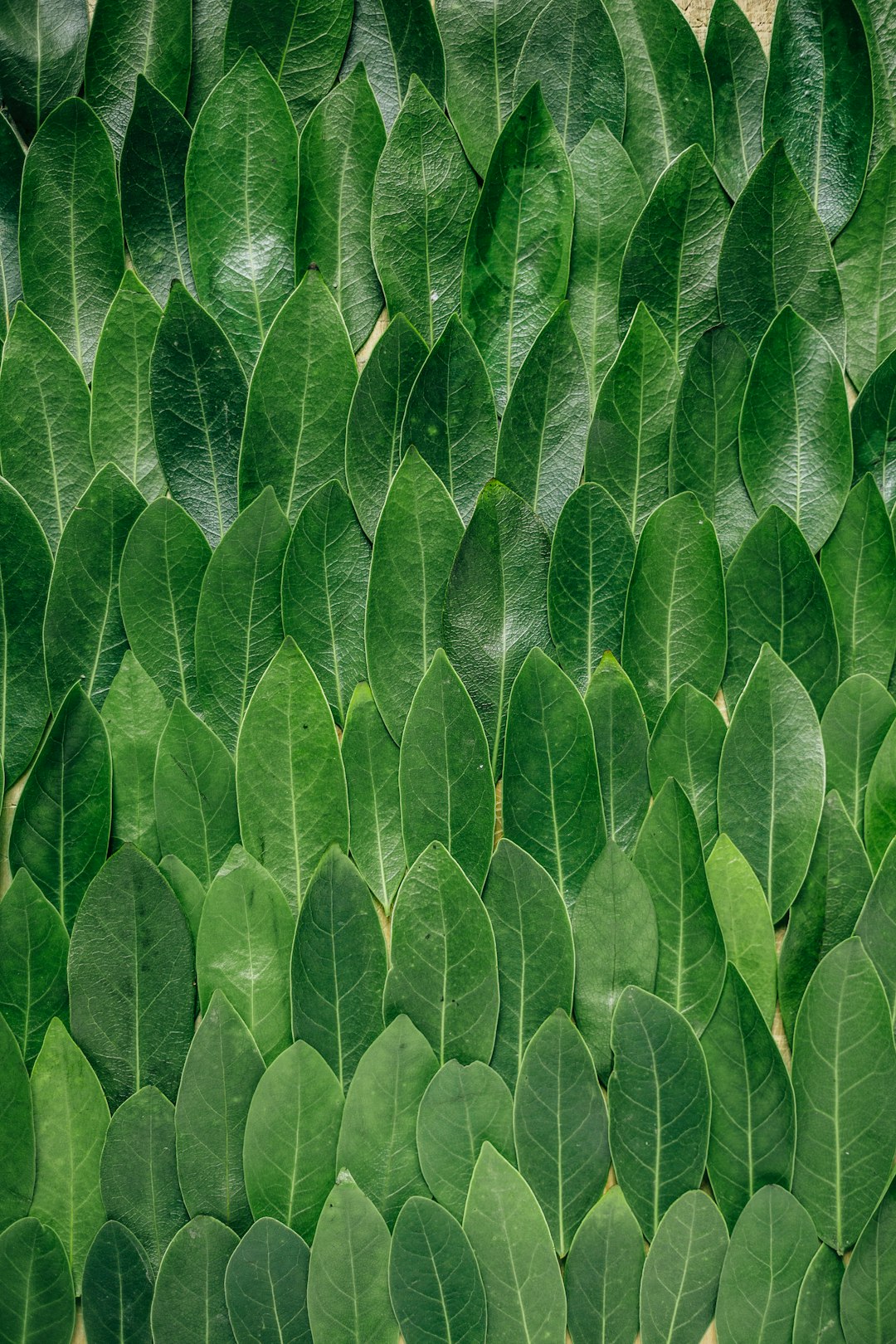
Types of Star Patterns
There are several ways to print star patterns in Java. Some of the common types include:
- Right-angled Triangle Pattern
- Inverted Right-angled Triangle Pattern
- Pyramid Pattern
- Diamond Pattern
- Hollow Square and Hollow Triangle Patterns
Example: Right-angled Triangle Pattern
The simplest form of a star pattern is the right-angled triangle. It involves using nested loops where the outer loop controls the number of rows, and the inner loop controls the number of columns.
Java Code for Right-angled Triangle
public class StarPattern {
public static void main(String[] args) {
int rows = 5;
for (int i = 1; i <= rows; i++) {
for (int j = 1; j <= i; j++) {
System.out.print("* ");
}
System.out.println();
}
}
}
Explanation:
- The outer loop runs from
1
to5
, indicating the number of rows. - The inner loop runs from
1
toi
and prints stars in each line, incrementing as the rows increase. - The
System.out.println()
ensures the cursor moves to the next line after printing each row.
When executed, this program produces the following output:
*
* *
* * *
* * * *
* * * * *
Example: Pyramid Star Pattern
A pyramid pattern is another interesting variation where spaces are used to center align the stars.
Java Code for a Pyramid Pattern
public class PyramidPattern {
public static void main(String[] args) {
int rows = 5;
for (int i = 1; i <= rows; i++) {
for (int j = 1; j <= rows - i; j++) {
System.out.print(" ");
}
for (int k = 1; k <= (2 * i - 1); k++) {
System.out.print("*");
}
System.out.println();
}
}
}
Expected output:
*
*
*
*
*
Image not found in postmeta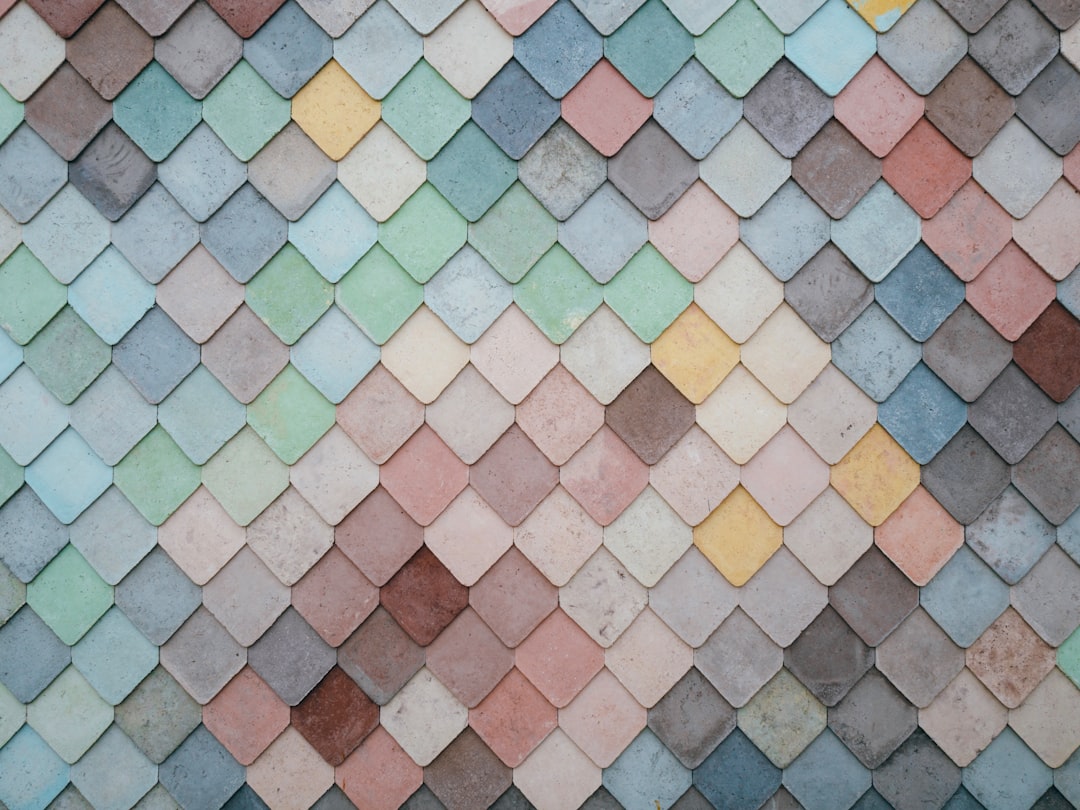
How Does the Star Algorithm Work?
The star algorithm works using the concept of nested loops:
- The outer loop determines the number of rows in the pattern.
- The inner loop handles the printing of spaces (for alignment) and stars.
- As the row index increases, the number of stars printed increases, creating the desired pattern.
Why Use Java Star Patterns?
While these patterns might seem simplistic, they serve multiple programming benefits:
- Improves logical thinking: Helps understand loop structures and nested conditions.
- Boosts problem-solving skills: Encourages breaking down a problem into smaller steps.
- Enhances coding confidence: A great exercise for beginners to practice and strengthen coding concepts.
- Used in job interviews: Many technical interviews feature pattern-related problems to assess a candidate’s ability to work with loops and conditions.
Conclusion
The Java star algorithm is a fundamental topic that helps in learning loop structures, nested iterations, and control statements. Whether you’re a beginner or someone looking to polish their logical approach to problem-solving, practicing star pattern programs is a great way to improve your coding skills.
Start practicing different star patterns and try modifying them to create new patterns! Understanding these simple exercises can build a strong foundation for more complex programming challenges.